owlcpp has three modules: rdf, io, and logic. Both io and logic modules depend on rdf, but can be built and used independently from each other.
Types
Type | Description |
---|---|
Triple_store | storage for RDF triples |
Ns_iri | namespace IRI |
Ns_id | a lightweight ID for namespace IRI |
Node | abstract class representing IRI, literal, or blank node |
Node_id | a lightweight node ID |
Doc_meta | OWL document description |
Doc_id | a lightweight document ID |
Triple | representation of RDF triple; three node IDs and a document ID |
boost::iterator_range<> | see Boost.Range |
Notation
Symbol | Description |
---|---|
ts | object of type Triple_store |
nsid | object of type Ns_id, namespace IRI ID |
nid | object of type Node_id |
did | object of type Doc_id |
ns_iri | object of type Ns_iri |
pref | object of type std::string representing namespace IRI prefix |
frag | object of type std::string representing IRI fragment identifier |
iri, iri1, iri2 | object of type std::string representing IRI |
n | unsigned integer |
t | object of type Triple |
rdf module
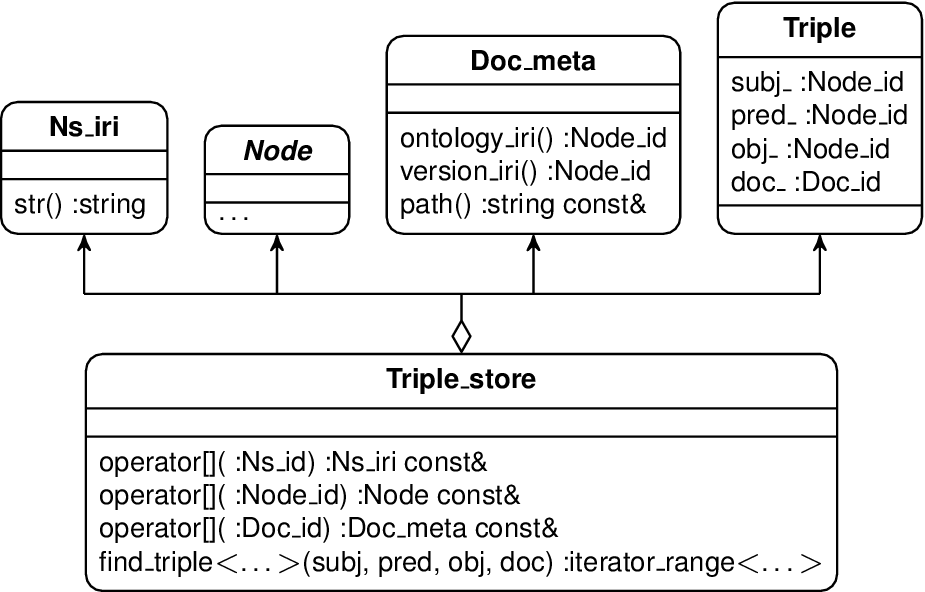
Triple store
owlcpp::Triple_store is defined in owlcpp/rdf/triple_store.hpp header.
Expression | Return type | Description |
---|---|---|
ts[nsid] | Ns_iri const& | return constant reference to corresponding namespace IRI. If nsid does not correspond to any namespace IRI, behavior is undefined. |
ts.at(nsid) | Ns_iri const& | return constant reference to corresponding namespace IRI. If nsid does not correspond to any namespace IRI, throw Map_ns::Err. |
ts.find(nsid) | Ns_iri const* | return constant pointer to corresponding namespace IRI class or 0. |
ts.prefix(nsid) | std::string | return namespace IRI prefix string or "" if no prefix was defined |
ts.find(ns_iri) | Ns_id const* | return pointer to namespace IRI ID or 0 if ns_iri is unknown |
ts.find_prefix(pref) | Ns_id const* | return pointer to namespace IRI ID or 0 if prefix pref is unknown |
ts.insert(ns_iri) | Ns_id | insert namespace IRI if it does not already exists, return the ID |
ts.insert_prefix(nid, pref) | Define or re-define prefix for a user-defined namespace IRI | |
ts[nid] | Node const& | return constant reference to corresponding Node. If nid does not correspond to any node, behavior is undefined. |
ts.at(nid) | Node const& | return constant reference to corresponding Node. If nid does not correspond to any node, throw Map_node::Err. |
ts.find(nid) | Node const* | return constant pointer to corresponding Node or 0. |
ts.find_node_iri(nsid, frag) | Node_id const* | find node IRI defined by namespace IRI ID, nsid, and fragment identifier, frag. Return constant pointer to the node ID or 0. |
ts.find_node_iri(iri) | Node_id const* | find IRI node defined by string iri. Return constant pointer to the node ID or 0. |
ts.find_literal(str, iri, lang) | Node_id const* | find literal node defined by its value string, str, datatype IRI, iri, and language lang. Return constant pointer to the node ID or 0. |
ts.find_blank(n, did) | Node_id const* | find blank node defined by its unique index, n, and document ID did. Return constant pointer to the node ID or 0. |
ts.insert_node_iri(nsid, frag) | Node_id | insert IRI node defined by namespace IRI ID, nsid, and fragment identifier, frag if not already present. Return corresponding node ID |
ts.insert_node_iri(iri) | Node_id | Insert IRI node defined by string iri if not already present. Return corresponding node ID. |
ts.insert_literal(str, nid, lang) | Node_id | insert literal node defined by its value string, str, ID for the node defining the datatype, nid, and language, lang if not already present. Return corresponding node ID. |
ts.insert_literal(str, iri, lang) | Node_id | insert literal node defined by its value string, str, datatype IRI, iri, and language, lang if not already present. Return corresponding node ID |
ts.insert_blank(n, did) | Node_id | insert blank node defined by its unique index, n, and document ID, did. Return corresponding node ID |
ts[did] | Doc_meta const& | return constant reference to corresponding document description. If did does not correspond to any document, behavior is undefined. |
ts.at(did) | Doc_meta const& | return constant reference to corresponding document description. If did does not correspond to any document, throw Map_doc::Err. |
ts.find(did) | Doc_meta const* | return constant pointer to corresponding document description or 0. |
ts.find_doc_iri(iri) | iterator_range<> | find documents with ontology IRI defined by iri. |
ts.find_doc_version(iri) | iterator_range<> | find documents with version IRI defined by iri. |
ts.insert_doc(nid1, path, nid2) | std::pair<Doc_id, bool> | insert OWL document info defined by it ontology IRI node ID, nid1, optional document filesystem path, path, and optional version IRI node ID nid2. |
ts.insert_doc(iri1, path, iri2) | std::pair<Doc_id, bool> | insert OWL document info defined by it ontology IRI, iri1, optional document filesystem path, path, and optional version IRI iri2. |
ts.find_triple(subj, pred, obj, doc) | iterator_range<> | Search triples by subject, predicate, object, or document IDs. Arguments subj, pred, obj can be of Node_id type; doc can be of Doc_id type. All of the arguments can be of Any type. |
ts.insert(t) | insert new triple |
io module
Catalog
owlcpp::Catalog is defined in owlcpp/io/catalog.hpp header.
Notation
Symbol | Description |
---|---|
cat | object of type Catalog |
bfs_path | object of type boost::filesystem::path; std::string and char const* are converted to it implicitly. |
Expression | Return type | Description |
---|---|---|
cat.size() | std::size_t | return number of documents described in catalog |
cat.begin() | Catalog::const_iterator | return iterator to first document description |
cat.end() | Catalog::const_iterator | return iterator past last document description |
cat[did] | Doc_meta const& | return constant reference to corresponding document description. If did does not correspond to any document, behavior is undefined. |
cat.at(did) | Doc_meta const& | return constant reference to corresponding document description. If did does not correspond to any document, throw Map_doc::Err. |
cat.find(did) | Doc_meta const* | return constant pointer to corresponding document description or 0. |
cat.find_doc_iri(iri) | iterator_range<> | find documents with ontology IRI defined by iri. |
cat.find_doc_version(iri) | iterator_range<> | find documents with version IRI defined by iri. |
cat.insert_doc(nid1, path, nid2) | std::pair<Doc_id, bool> | insert OWL document info defined by it ontology IRI node ID, nid1, optional document filesystem path, path, and optional version IRI node ID nid2. |
cat.insert_doc(iri1, path, iri2) | std::pair<Doc_id, bool> | insert OWL document info defined by it ontology IRI, iri1, optional document filesystem path, path, and optional version IRI iri2. Return document ID and whether it was actually inserted. |
cat.ontology_iri_str(did) | std::string | return ontology IRI string |
cat.version_iri_str(did) | std::string | return version IRI string |
add(cat, bfs_path, recurse) | std::size_t | determine OntologyIRI and VersionIRI of ontology document(s) pointed by filesystem path bfs_path and add them to the catalog. If path is a directory, an attempt is made to parse every file located in it. Files that fail to parse are ignored. If recurse is true, subdirectories of path are also searched. Return number of documents inserted. |
io methods
io module methods are declared in owlcpp/io/input.hpp header.
Expression | Return type | Description |
---|---|---|
load(istream, ts, path) | Load one ontology document from std::istream to triple store. Optional path is used as a reference only. Imported ontologies are not loaded. Throw Input_err if input ontology contains an error or an ontology with the same ID is already in the triple store. If an exception is thrown, the destination triple store remains unchanged. | |
load(istream, ts, cat, path) | Load ontology document from std::istream and its imports to triple store. Optional path is used as a reference only. Imported ontologies are resolved against cat. Throw Input_err if input ontology contains an error or an ontology with the same ID is already in the triple store. If an exception is thrown, the destination triple store remains unchanged. | |
load_file(bfs_path, ts) | Load one ontology document from filesystem to triple store. Imported ontologies are not loaded. Throw Input_err if input ontology contains an error or an ontology with the same ID is already in the triple store. If an exception is thrown, the destination triple store remains unchanged. | |
load(bfs_path, ts, cat) | Load ontology document from filesystem and its imports to triple store. Imported ontologies are resolved against cat. Throw Input_err if input ontology contains an error or an ontology with the same ID is already in the triple store. If an exception is thrown, the destination triple store remains unchanged. | |
load_iri(iri, ts, cat) | Load ontology identified by versionIRI or ontologyIRI and its imports. Ontology IRIs are resolved against cat. Throw Input_err if input ontology contains an error or an ontology with the same ID is already in the triple store. If an exception is thrown, the destination triple store remains unchanged. |
logic module
logic module methods are declared in owlcpp/logic/triple_to_fact.hpp header.
Types
Type | Description |
---|---|
ReasoningKernel | FaCT++ reasoning kernel |
Notation
Symbol | Description |
---|---|
kernel | object of type ReasoningKernel |
Expression | Return type | Description |
---|---|---|
submit(ts, kernel, strict, diagnose) | std::size_t | Convert RDF triples from the triple store to axioms and submit them to reasoning kernel. If strict is true (default), all requirements of OWL 2 Mapping to RDF Graphs are enforced. Currently, strict==false results in same behavior. If diagnose==true, peform reasoner classification after every axiom to diagnose errors. Return number of axioms submitted. |
submit(r, ts, kernel, strict, diagnose) | std::size_t | Convert RDF triples from triple range r to axioms and submit them to reasoning kernel. If strict is true (default), all requirements of OWL 2 Mapping to RDF Graphs are enforced. Currently, strict==false results in same behavior. If diagnose==true, peform reasoner classification after every axiom to diagnose errors. Return number of axioms submitted. |